How to Read Value From Array Object in Javascript
Affiliate ivData Structures: Objects and Arrays
On 2 occasions I take been asked, 'Pray, Mr. Babbage, if you lot put into the machine incorrect figures, will the correct answers come up out?' [...] I am not able rightly to apprehend the kind of confusion of ideas that could provoke such a question.
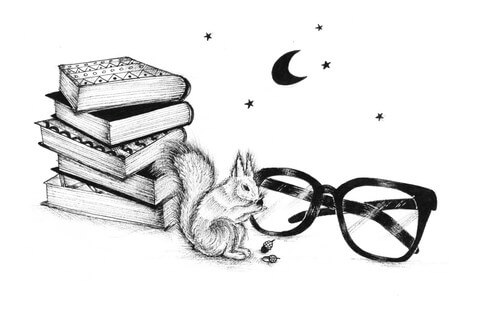
Numbers, Booleans, and strings are the atoms that data structures are built from. Many types of information require more than than one atom, though. Objects permit us to grouping values—including other objects—to build more than circuitous structures.
The programs we have built so far have been limited by the fact that they were operating just on uncomplicated data types. This affiliate volition introduce basic data structures. Past the end of it, you'll know plenty to beginning writing useful programs.
The chapter will work through a more or less realistic programming instance, introducing concepts as they utilize to the problem at hand. The example code volition often build on functions and bindings that were introduced earlier in the text.
The weresquirrel
Every now and then, usually between 8 p.m. and ten p.m., Jacques finds himself transforming into a small furry rodent with a bushy tail.
On one paw, Jacques is quite glad that he doesn't have archetype lycanthropy. Turning into a squirrel does cause fewer problems than turning into a wolf. Instead of having to worry nigh accidentally eating the neighbor (that would be awkward), he worries nigh being eaten by the neighbor'due south cat. After two occasions where he woke up on a precariously thin branch in the crown of an oak, naked and disoriented, he has taken to locking the doors and windows of his room at dark and putting a few walnuts on the floor to keep himself busy.
That takes intendance of the cat and tree problems. But Jacques would prefer to get rid of his condition entirely. The irregular occurrences of the transformation brand him suspect that they might be triggered by something. For a while, he believed that it happened only on days when he had been virtually oak trees. Simply avoiding oak trees did not stop the problem.
Switching to a more scientific approach, Jacques has started keeping a daily log of everything he does on a given day and whether he changed form. With this information he hopes to narrow downward the conditions that trigger the transformations.
The first affair he needs is a data structure to store this information.
Data sets
To work with a chunk of digital information, we'll commencement accept to observe a way to represent it in our automobile's memory. Say, for case, that we want to stand for a drove of the numbers 2, iii, v, vii, and 11.
Nosotros could get creative with strings—after all, strings tin take whatever length, and so we tin can put a lot of information into them—and utilise "ii 3 5 seven 11"
as our representation. Merely this is awkward. You'd have to somehow extract the digits and convert them back to numbers to access them.
Fortunately, JavaScript provides a data type specifically for storing sequences of values. Information technology is called an array and is written equally a listing of values between foursquare brackets, separated by commas.
let listOfNumbers = [2, 3, five, 7, 11]; console.log(listOfNumbers[2]); console.log(listOfNumbers[0]); panel.log(listOfNumbers[2 - one]);
The notation for getting at the elements within an array also uses square brackets. A pair of square brackets immediately subsequently an expression, with another expression inside of them, volition look up the element in the left-paw expression that corresponds to the index given by the expression in the brackets.
The first index of an assortment is naught, not one. And so the get-go element is retrieved with listOfNumbers[0]
. Zip-based counting has a long tradition in technology and in certain ways makes a lot of sense, but it takes some getting used to. Think of the index as the amount of items to skip, counting from the start of the array.
Backdrop
We've seen a few suspicious-looking expressions like myString.length
(to get the length of a string) and Math.max
(the maximum function) in past chapters. These are expressions that access a holding of some value. In the first case, we access the length
property of the value in myString
. In the second, nosotros access the holding named max
in the Math
object (which is a collection of mathematics-related constants and functions).
About all JavaScript values accept properties. The exceptions are null
and undefined
. If you lot endeavor to admission a property on i of these nonvalues, you become an error.
null.length;
The 2 main means to admission properties in JavaScript are with a dot and with foursquare brackets. Both value.10
and value[x]
access a property on value
—simply non necessarily the same property. The difference is in how x
is interpreted. When using a dot, the word afterwards the dot is the literal name of the property. When using square brackets, the expression between the brackets is evaluated to get the belongings name. Whereas value.10
fetches the property of value
named "x", value[ten]
tries to evaluate the expression x
and uses the result, converted to a string, as the property proper name.
So if you know that the belongings you are interested in is chosen colour, you say value.color
. If you want to extract the holding named by the value held in the binding i
, you lot say value[i]
. Belongings names are strings. They tin be whatsoever string, only the dot annotation works but with names that await like valid bounden names. So if yous want to access a holding named 2 or John Doe, yous must use square brackets: value[ii]
or value["John Doe"]
.
The elements in an array are stored as the array's properties, using numbers every bit property names. Because you can't apply the dot note with numbers and usually want to apply a binding that holds the index anyhow, you have to use the bracket note to get at them.
The length
property of an array tells us how many elements it has. This property proper name is a valid binding name, and nosotros know its name in accelerate, and then to find the length of an array, you typically write array.length
considering that'due south easier to write than array["length"]
.
Methods
Both cord and assortment values incorporate, in add-on to the length
property, a number of properties that hold function values.
let doh = "Doh"; console.log(typeof doh.toUpperCase); console.log(doh.toUpperCase());
Every cord has a toUpperCase
belongings. When chosen, it volition return a copy of the string in which all letters have been converted to capital. There is also toLowerCase
, going the other style.
Interestingly, even though the call to toUpperCase
does not laissez passer whatsoever arguments, the function somehow has access to the cord "Doh"
, the value whose property we called. How this works is described in Chapter 6.
Properties that contain functions are generally called methods of the value they belong to, equally in "toUpperCase
is a method of a string".
This instance demonstrates two methods you can use to manipulate arrays:
permit sequence = [1, 2, 3]; sequence.push(4); sequence.push(5); console.log(sequence); console.log(sequence.pop()); panel.log(sequence);
The push
method adds values to the end of an array, and the popular
method does the opposite, removing the terminal value in the array and returning it.
These somewhat silly names are the traditional terms for operations on a stack. A stack, in programming, is a data structure that allows y'all to push values into it and pop them out again in the opposite guild so that the thing that was added terminal is removed first. These are common in programming—you might call back the function call stack from the previous chapter, which is an example of the same idea.
Objects
Back to the weresquirrel. A fix of daily log entries can exist represented every bit an array. But the entries practise non consist of just a number or a string—each entry needs to store a list of activities and a Boolean value that indicates whether Jacques turned into a squirrel or not. Ideally, we would like to grouping these together into a single value and and then put those grouped values into an assortment of log entries.
Values of the type object are arbitrary collections of properties. One way to create an object is by using braces as an expression.
permit day1 = { squirrel: false, events: ["work", "touched tree", "pizza", "running"] }; console.log(day1.squirrel); console.log(day1.wolf); day1.wolf = false; panel.log(day1.wolf);
Inside the braces, in that location is a list of properties separated by commas. Each property has a proper name followed by a colon and a value. When an object is written over multiple lines, indenting it like in the example helps with readability. Backdrop whose names aren't valid binding names or valid numbers take to be quoted.
let descriptions = { piece of work: "Went to work", "touched tree": "Touched a tree" };
This ways that braces take two meanings in JavaScript. At the offset of a statement, they start a block of statements. In any other position, they depict an object. Fortunately, it is rarely useful to start a statement with an object in braces, and so the ambiguity betwixt these two is not much of a trouble.
Reading a property that doesn't be will give you the value undefined
.
It is possible to assign a value to a belongings expression with the =
operator. This will supplant the property'southward value if it already existed or create a new property on the object if it didn't.
To briefly return to our tentacle model of bindings—property bindings are similar. They grasp values, just other bindings and backdrop might be holding onto those same values. You may recollect of objects as octopuses with any number of tentacles, each of which has a proper name tattooed on it.
The delete
operator cuts off a tentacle from such an octopus. Information technology is a unary operator that, when applied to an object property, will remove the named property from the object. This is not a common thing to do, but it is possible.
allow anObject = {left: one, right: 2}; console.log(anObject.left); delete anObject.left; console.log(anObject.left); console.log("left" in anObject); console.log("right" in anObject);
The binary in
operator, when applied to a string and an object, tells you whether that object has a property with that proper noun. The difference between setting a property to undefined
and actually deleting it is that, in the first case, the object still has the belongings (it merely doesn't have a very interesting value), whereas in the 2d case the property is no longer present and in
will return false
.
To find out what properties an object has, you tin utilise the Object.keys
office. You give it an object, and information technology returns an assortment of strings—the object'due south belongings names.
console.log(Object.keys({x: 0, y: 0, z: 2}));
At that place's an Object.assign
role that copies all properties from i object into another.
let objectA = {a: ane, b: 2}; Object.assign(objectA, {b: iii, c: 4}); console.log(objectA);
Arrays, then, are just a kind of object specialized for storing sequences of things. If yous evaluate typeof []
, it produces "object"
. Yous can see them as long, flat octopuses with all their tentacles in a bang-up row, labeled with numbers.
We will represent the journal that Jacques keeps as an array of objects.
let journal = [ {events: ["work", "touched tree", "pizza", "running", "television"], squirrel: false}, {events: ["work", "ice cream", "cauliflower", "lasagna", "touched tree", "brushed teeth"], squirrel: false}, {events: ["weekend", "cycling", "break", "peanuts", "beer"], squirrel: true}, ];
Mutability
We volition get to actual programming real soon now. First at that place's one more piece of theory to understand.
Nosotros saw that object values tin be modified. The types of values discussed in earlier chapters, such as numbers, strings, and Booleans, are all immutable—it is impossible to alter values of those types. Yous can combine them and derive new values from them, but when you take a specific cord value, that value volition always remain the same. The text inside information technology cannot be changed. If you accept a string that contains "cat"
, it is not possible for other lawmaking to change a character in your string to make information technology spell "rat"
.
Objects work differently. You can change their properties, causing a unmarried object value to have different content at dissimilar times.
When nosotros have 2 numbers, 120 and 120, nosotros tin consider them precisely the same number, whether or not they refer to the same physical bits. With objects, there is a difference betwixt having ii references to the same object and having two different objects that contain the same properties. Consider the following code:
let object1 = {value: 10}; let object2 = object1; let object3 = {value: 10}; console.log(object1 == object2); console.log(object1 == object3); object1.value = 15; console.log(object2.value); console.log(object3.value);
The object1
and object2
bindings grasp the same object, which is why changing object1
likewise changes the value of object2
. They are said to have the aforementioned identity. The binding object3
points to a different object, which initially contains the same backdrop equally object1
but lives a carve up life.
Bindings tin can also exist changeable or abiding, merely this is split from the mode their values acquit. Fifty-fifty though number values don't change, you can use a let
binding to proceed rail of a changing number by changing the value the binding points at. Similarly, though a const
binding to an object can itself non be changed and will keep to point at the same object, the contents of that object might change.
const score = {visitors: 0, home: 0}; score.visitors = 1; score = {visitors: i, home: 1};
When yous compare objects with JavaScript'southward ==
operator, it compares past identity: it will produce true
only if both objects are precisely the same value. Comparing different objects volition return false
, even if they have identical properties. At that place is no "deep" comparing operation built into JavaScript, which compares objects past contents, but information technology is possible to write information technology yourself (which is one of the exercises at the end of this chapter).
The lycanthrope's log
And then, Jacques starts upwards his JavaScript interpreter and sets up the environment he needs to keep his journal.
allow journal = []; function addEntry(events, squirrel) { periodical.push({events, squirrel}); }
Note that the object added to the journal looks a niggling odd. Instead of declaring properties similar events: events
, information technology just gives a property proper noun. This is shorthand that means the same thing—if a property proper noun in brace notation isn't followed by a value, its value is taken from the binding with the same name.
So then, every evening at x p.thou.—or sometimes the next morning, after climbing downward from the superlative shelf of his bookcase—Jacques records the twenty-four hours.
addEntry(["work", "touched tree", "pizza", "running", "television"], fake); addEntry(["piece of work", "ice cream", "cauliflower", "lasagna", "touched tree", "brushed teeth"], simulated); addEntry(["weekend", "cycling", "break", "peanuts", "beer"], true);
Once he has enough information points, he intends to use statistics to discover out which of these events may be related to the squirrelifications.
Correlation is a measure of dependence between statistical variables. A statistical variable is not quite the aforementioned as a programming variable. In statistics you typically have a set of measurements, and each variable is measured for every measurement. Correlation between variables is normally expressed every bit a value that ranges from -one to one. Zero correlation ways the variables are not related. A correlation of 1 indicates that the two are perfectly related—if you know one, you lot also know the other. Negative one as well means that the variables are perfectly related but that they are opposites—when one is true, the other is fake.
To compute the measure of correlation between two Boolean variables, nosotros can utilise the phi coefficient (ϕ). This is a formula whose input is a frequency table containing the number of times the different combinations of the variables were observed. The output of the formula is a number between -one and 1 that describes the correlation.
We could take the issue of eating pizza and put that in a frequency tabular array like this, where each number indicates the amount of times that combination occurred in our measurements:
If we telephone call that tabular array northward, we can compute ϕ using the following formula:
ϕ = | n 11 due north 00 − n x n 01 √ due north i• n 0• north •1 n •0 |
(If at this point you're putting the book down to focus on a terrible flashback to 10th form math course—hold on! I exercise not intend to torture yous with countless pages of cryptic notation—it's merely this one formula for now. And fifty-fifty with this one, all we do is turn information technology into JavaScript.)
The notation n 01 indicates the number of measurements where the first variable (squirrelness) is false (0) and the 2d variable (pizza) is true (1). In the pizza table, n 01 is 9.
The value due north 1• refers to the sum of all measurements where the first variable is true, which is v in the example table. Besides, north •0 refers to the sum of the measurements where the second variable is false.
So for the pizza table, the office higher up the division line (the dividend) would be one×76−four×9 = 40, and the part below it (the divisor) would be the square root of 5×85×10×80, or √340000. This comes out to ϕ ≈ 0.069, which is tiny. Eating pizza does not appear to accept influence on the transformations.
Calculating correlation
We can represent a two-by-two table in JavaScript with a four-element array ([76, nine, four, i]
). Nosotros could also use other representations, such equally an array containing two 2-element arrays ([[76, 9], [4, 1]]
) or an object with property names like "11"
and "01"
, only the flat array is simple and makes the expressions that access the tabular array pleasantly short. We'll interpret the indices to the array equally two-scrap binary numbers, where the leftmost (most significant) digit refers to the squirrel variable and the rightmost (least significant) digit refers to the consequence variable. For example, the binary number 10
refers to the case where Jacques did plow into a squirrel, just the result (say, "pizza") didn't occur. This happened iv times. And since binary 10
is 2 in decimal notation, we volition shop this number at index ii of the array.
This is the function that computes the ϕ coefficient from such an array:
function phi(table) { return (table[3] * tabular array[0] - table[2] * table[1]) / Math.sqrt((table[ii] + table[3]) * (table[0] + table[i]) * (table[one] + table[3]) * (table[0] + table[2])); } console.log(phi([76, ix, 4, ane]));
This is a direct translation of the ϕ formula into JavaScript. Math.sqrt
is the square root function, as provided by the Math
object in a standard JavaScript environs. We accept to add two fields from the table to get fields like due north1• considering the sums of rows or columns are not stored straight in our data construction.
Jacques kept his periodical for three months. The resulting data set is available in the coding sandbox for this chapter, where it is stored in the Journal
binding and in a downloadable file.
To extract a two-past-two table for a specific event from the journal, we must loop over all the entries and tally how many times the event occurs in relation to squirrel transformations.
office tableFor(outcome, journal) { permit table = [0, 0, 0, 0]; for (let i = 0; i < journal.length; i ++) { let entry = periodical[i], index = 0; if (entry.events.includes(outcome)) index += i; if (entry.squirrel) index += 2; table[index] += 1; } return table; } console.log(tableFor("pizza", JOURNAL));
Arrays have an includes
method that checks whether a given value exists in the array. The function uses that to decide whether the issue proper noun information technology is interested in is part of the outcome list for a given day.
The torso of the loop in tableFor
figures out which box in the table each journal entry falls into by checking whether the entry contains the specific event information technology'due south interested in and whether the result happens alongside a squirrel incident. The loop then adds one to the right box in the table.
We now have the tools we need to compute individual correlations. The only step remaining is to find a correlation for every blazon of effect that was recorded and see whether anything stands out.
Array loops
In the tableFor
part, there's a loop like this:
for (let i = 0; i < JOURNAL.length; i ++) { let entry = JOURNAL[i]; }
This kind of loop is common in classical JavaScript—going over arrays one element at a time is something that comes up a lot, and to practice that yous'd run a counter over the length of the array and choice out each chemical element in plow.
There is a simpler way to write such loops in modern JavaScript.
for (permit entry of JOURNAL) { console.log(`${ entry.events.length } events.`); }
When a for
loop looks similar this, with the word of
subsequently a variable definition, information technology volition loop over the elements of the value given later of
. This works not simply for arrays but besides for strings and some other data structures. We'll discuss how it works in Affiliate half dozen.
The final analysis
We demand to compute a correlation for every type of event that occurs in the data set. To do that, nosotros starting time demand to find every type of upshot.
function journalEvents(journal) { let events = []; for (let entry of journal) { for (allow effect of entry.events) { if (! events.includes(event)) { events.push(event); } } } return events; } console.log(journalEvents(JOURNAL));
Past going over all the events and calculation those that aren't already in there to the events
array, the role collects every blazon of event.
Using that, we tin meet all the correlations.
for (let effect of journalEvents(JOURNAL)) { console.log(upshot + ":", phi(tableFor(result, Journal))); }
Most correlations seem to lie close to nada. Eating carrots, breadstuff, or pudding apparently does non trigger squirrel-lycanthropy. It does seem to occur somewhat more often on weekends. Permit's filter the results to show but correlations greater than 0.1 or less than -0.1.
for (let event of journalEvents(Journal)) { permit correlation = phi(tableFor(event, JOURNAL)); if (correlation > 0.1 | | correlation < - 0.ane) { console.log(result + ":", correlation); } }
Aha! At that place are two factors with a correlation that's conspicuously stronger than the others. Eating peanuts has a strong positive effect on the take chances of turning into a squirrel, whereas brushing his teeth has a significant negative upshot.
Interesting. Let's attempt something.
for (let entry of Journal) { if (entry.events.includes("peanuts") & & ! entry.events.includes("brushed teeth")) { entry.events.push button("peanut teeth"); } } console.log(phi(tableFor("peanut teeth", Journal)));
That's a strong result. The phenomenon occurs precisely when Jacques eats peanuts and fails to brush his teeth. If merely he weren't such a slob about dental hygiene, he'd accept never fifty-fifty noticed his affliction.
Knowing this, Jacques stops eating peanuts altogether and finds that his transformations don't come up dorsum.
For a few years, things become great for Jacques. But at some signal he loses his task. Because he lives in a nasty country where having no job means having no medical services, he is forced to take employment with a circus where he performs as The Incredible Squirrelman, stuffing his mouth with peanut butter before every show.
I twenty-four hours, fed up with this sorry existence, Jacques fails to alter dorsum into his human grade, hops through a scissure in the circus tent, and vanishes into the forest. He is never seen again.
Further arrayology
Before finishing the affiliate, I desire to innovate y'all to a few more object-related concepts. I'll beginning past introducing some more often than not useful array methods.
We saw push
and popular
, which add and remove elements at the end of an array, earlier in this chapter. The corresponding methods for calculation and removing things at the beginning of an array are called unshift
and shift
.
let todoList = []; function recall(task) { todoList.push(task); } function getTask() { return todoList.shift(); } office rememberUrgently(task) { todoList.unshift(task); }
That plan manages a queue of tasks. You add together tasks to the finish of the queue by calling call back("groceries")
, and when you're set to do something, you call getTask()
to get (and remove) the front particular from the queue. The rememberUrgently
function also adds a task but adds it to the front instead of the back of the queue.
To search for a specific value, arrays provide an indexOf
method. The method searches through the array from the start to the end and returns the index at which the requested value was plant—or -ane if it wasn't establish. To search from the end instead of the showtime, there's a like method called lastIndexOf
.
console.log([1, 2, iii, 2, one].indexOf(2)); console.log([i, 2, iii, ii, 1].lastIndexOf(2));
Both indexOf
and lastIndexOf
take an optional 2d argument that indicates where to start searching.
Some other central assortment method is slice
, which takes get-go and stop indices and returns an assortment that has only the elements between them. The showtime index is inclusive, the end alphabetize exclusive.
console.log([0, 1, 2, 3, 4].slice(2, 4)); console.log([0, 1, ii, 3, 4].piece(2));
When the end index is not given, slice
will take all of the elements after the get-go alphabetize. You tin also omit the start index to copy the entire array.
The concat
method tin be used to gum arrays together to create a new array, similar to what the +
operator does for strings.
The following case shows both concat
and slice
in action. It takes an assortment and an alphabetize, and information technology returns a new array that is a copy of the original array with the element at the given index removed.
function remove(array, index) { return assortment.slice(0, index) .concat(assortment.slice(index + 1)); } console.log(remove(["a", "b", "c", "d", "e"], ii));
If you pass concat
an argument that is non an array, that value will be added to the new array equally if information technology were a 1-element array.
Strings and their properties
We can read properties similar length
and toUpperCase
from string values. Only if you lot attempt to add a new property, it doesn't stick.
allow kim = "Kim"; kim.age = 88; panel.log(kim.age);
Values of type string, number, and Boolean are non objects, and though the language doesn't mutter if you endeavor to fix new properties on them, it doesn't really shop those backdrop. Equally mentioned earlier, such values are immutable and cannot be inverse.
Simply these types do have built-in properties. Every string value has a number of methods. Some very useful ones are piece
and indexOf
, which resemble the array methods of the same name.
panel.log("coconuts".slice(4, 7)); console.log("kokosnoot".indexOf("u"));
One difference is that a string's indexOf
tin search for a string containing more than i graphic symbol, whereas the respective array method looks only for a single element.
console.log("one ii three".indexOf("ee"));
The trim
method removes whitespace (spaces, newlines, tabs, and like characters) from the start and end of a string.
console.log(" okay \north ".trim());
The zeroPad
function from the previous chapter also exists as a method. It is chosen padStart
and takes the desired length and padding character as arguments.
console.log(String(half-dozen).padStart(3, "0"));
You lot can divide a string on every occurrence of another cord with split
and join it again with bring together
.
let judgement = "Secretarybirds specialize in stomping"; let words = sentence.split(" "); console.log(words); console.log(words.join(". "));
A string can be repeated with the repeat
method, which creates a new string containing multiple copies of the original string, glued together.
console.log("LA".repeat(3));
We have already seen the cord type's length
property. Accessing the individual characters in a string looks like accessing assortment elements (with a caveat that nosotros'll discuss in Chapter v).
let string = "abc"; console.log(string.length); console.log(cord[1]);
Rest parameters
It tin can be useful for a office to accept whatsoever number of arguments. For example, Math.max
computes the maximum of all the arguments it is given.
To write such a function, you put three dots earlier the function's last parameter, like this:
function max( numbers) { let issue = - Infinity; for (let number of numbers) { if (number > result) result = number; } return event; } console.log(max(iv, 1, 9, - ii));
When such a function is called, the balance parameter is jump to an array containing all further arguments. If in that location are other parameters earlier information technology, their values aren't part of that array. When, every bit in max
, it is the only parameter, it will hold all arguments.
You can employ a like three-dot notation to call a role with an assortment of arguments.
let numbers = [5, one, 7]; panel.log(max( numbers));
This "spreads" out the array into the part call, passing its elements every bit separate arguments. It is possible to include an array like that along with other arguments, every bit in max(9, .
.
Foursquare bracket array notation similarly allows the triple-dot operator to spread another assortment into the new array.
let words = ["never", "fully"]; console.log(["volition", words, "understand"]);
The Math object
As we've seen, Math
is a grab bag of number-related utility functions, such equally Math.max
(maximum), Math.min
(minimum), and Math.sqrt
(square root).
The Math
object is used equally a container to group a agglomeration of related functionality. There is only one Math
object, and information technology is near never useful as a value. Rather, information technology provides a namespace then that all these functions and values do not have to be global bindings.
Having also many global bindings "pollutes" the namespace. The more names have been taken, the more than probable you lot are to accidentally overwrite the value of some existing bounden. For example, it's not unlikely to want to name something max
in one of your programs. Since JavaScript's built-in max
office is tucked safely within the Math
object, we don't have to worry about overwriting it.
Many languages will stop y'all, or at least warn yous, when you are defining a bounden with a proper noun that is already taken. JavaScript does this for bindings yous declared with let
or const
only—perversely—not for standard bindings nor for bindings declared with var
or function
.
Back to the Math
object. If you need to do trigonometry, Math
can aid. It contains cos
(cosine), sin
(sine), and tan
(tangent), as well as their inverse functions, acos
, asin
, and atan
, respectively. The number π (pi)—or at least the closest approximation that fits in a JavaScript number—is available as Math.PI
. There is an old programming tradition of writing the names of constant values in all caps.
function randomPointOnCircle(radius) { let bending = Math.random() * 2 * Math.PI; render {x: radius * Math.cos(angle), y: radius * Math.sin(angle)}; } console.log(randomPointOnCircle(ii));
If sines and cosines are not something you are familiar with, don't worry. When they are used in this book, in Chapter xiv, I'll explain them.
The previous case used Math.random
. This is a office that returns a new pseudorandom number between zero (inclusive) and one (sectional) every time y'all telephone call information technology.
console.log(Math.random()); console.log(Math.random()); panel.log(Math.random());
Though computers are deterministic machines—they always react the aforementioned mode if given the same input—it is possible to have them produce numbers that appear random. To do that, the machine keeps some hidden value, and whenever you enquire for a new random number, it performs complicated computations on this hidden value to create a new value. It stores a new value and returns some number derived from information technology. That way, it tin produce always new, hard-to-predict numbers in a way that seems random.
If we want a whole random number instead of a partial i, we can use Math.floor
(which rounds downwardly to the nearest whole number) on the effect of Math.random
.
console.log(Math.floor(Math.random() * 10));
Multiplying the random number past 10 gives united states a number greater than or equal to 0 and below 10. Since Math.floor
rounds down, this expression will produce, with equal adventure, whatever number from 0 through nine.
There are also the functions Math.ceil
(for "ceiling", which rounds up to a whole number), Math.circular
(to the nearest whole number), and Math.abs
, which takes the absolute value of a number, meaning information technology negates negative values but leaves positive ones as they are.
Destructuring
Let's go dorsum to the phi
function for a moment.
office phi(table) { render (table[3] * table[0] - table[ii] * table[1]) / Math.sqrt((table[2] + table[iii]) * (table[0] + tabular array[1]) * (table[i] + table[3]) * (table[0] + tabular array[2])); }
One of the reasons this function is awkward to read is that we take a binding pointing at our assortment, only we'd much prefer to take bindings for the elements of the array, that is, let n00 = table[0]
and then on. Fortunately, in that location is a succinct style to do this in JavaScript.
function phi([n00, n01, n10, n11]) { return (n11 * n00 - n10 * n01) / Math.sqrt((n10 + n11) * (n00 + n01) * (n01 + n11) * (n00 + n10)); }
This also works for bindings created with allow
, var
, or const
. If you know the value yous are binding is an array, you tin can use square brackets to "look within" of the value, binding its contents.
A similar pull a fast one on works for objects, using braces instead of square brackets.
let {name} = {name: "Faraji", historic period: 23}; console.log(proper name);
Annotation that if you endeavour to destructure null
or undefined
, you get an error, much as you lot would if you directly attempt to access a property of those values.
JSON
Because properties merely grasp their value, rather than incorporate it, objects and arrays are stored in the reckoner'due south retentivity as sequences of bits holding the addresses—the identify in retention—of their contents. And so an array with some other array inside of information technology consists of (at least) one memory region for the inner array, and another for the outer array, containing (among other things) a binary number that represents the position of the inner assortment.
If yous desire to save data in a file for later or send information technology to another computer over the network, yous have to somehow convert these tangles of memory addresses to a description that can be stored or sent. You could ship over your entire figurer memory along with the accost of the value you're interested in, I suppose, but that doesn't seem like the all-time approach.
What we tin practice is serialize the data. That means it is converted into a flat description. A popular serialization format is called JSON (pronounced "Jason"), which stands for JavaScript Object Notation. Information technology is widely used as a data storage and communication format on the Spider web, fifty-fifty in languages other than JavaScript.
JSON looks similar to JavaScript'south way of writing arrays and objects, with a few restrictions. All property names have to be surrounded by double quotes, and just uncomplicated information expressions are allowed—no part calls, bindings, or anything that involves actual ciphering. Comments are not allowed in JSON.
A journal entry might look similar this when represented as JSON data:
{ "squirrel": fake, "events": ["work", "touched tree", "pizza", "running"] }
JavaScript gives united states of america the functions JSON.stringify
and JSON.parse
to convert data to and from this format. The first takes a JavaScript value and returns a JSON-encoded string. The second takes such a cord and converts it to the value it encodes.
permit string = JSON.stringify({squirrel: false, events: ["weekend"]}); console.log(string); panel.log(JSON.parse(cord).events);
Summary
Objects and arrays (which are a specific kind of object) provide means to grouping several values into a single value. Conceptually, this allows us to put a bunch of related things in a handbag and run around with the handbag, instead of wrapping our arms around all of the private things and trying to hold on to them separately.
Most values in JavaScript take backdrop, the exceptions beingness null
and undefined
. Properties are accessed using value.prop
or value["prop"]
. Objects tend to utilize names for their properties and store more or less a fixed prepare of them. Arrays, on the other hand, usually contain varying amounts of conceptually identical values and use numbers (starting from 0) equally the names of their backdrop.
There are some named properties in arrays, such as length
and a number of methods. Methods are functions that alive in properties and (unremarkably) human activity on the value they are a property of.
Yous can iterate over arrays using a special kind of for
loop—for (let element of array)
.
Exercises
The sum of a range
The introduction of this book alluded to the following every bit a nice mode to compute the sum of a range of numbers:
console.log(sum(range(1, 10)));
Write a range
function that takes two arguments, start
and terminate
, and returns an array containing all the numbers from beginning
upward to (and including) terminate
.
Next, write a sum
role that takes an array of numbers and returns the sum of these numbers. Run the example program and see whether it does indeed return 55.
As a bonus assignment, alter your range
part to take an optional third argument that indicates the "pace" value used when building the array. If no stride is given, the elements go up by increments of one, respective to the old behavior. The function phone call range(1, x, ii)
should return [one, 3, five, 7, 9]
. Make sure it also works with negative step values and so that range(five, two, -1)
produces [5, four, 3, 2]
.
console.log(range(1, 10)); console.log(range(5, 2, - 1)); console.log(sum(range(1, 10)));
Building upwards an array is virtually easily done by starting time initializing a binding to []
(a fresh, empty array) and repeatedly calling its button
method to add a value. Don't forget to return the assortment at the terminate of the function.
Since the end boundary is inclusive, you'll need to use the <=
operator rather than <
to cheque for the end of your loop.
The step parameter can be an optional parameter that defaults (using the =
operator) to 1.
Having range
sympathize negative pace values is probably best washed by writing two separate loops—one for counting upwardly and 1 for counting down—because the comparison that checks whether the loop is finished needs to be >=
rather than <=
when counting downward.
It might also be worthwhile to employ a unlike default step, namely, -1, when the end of the range is smaller than the start. That way, range(5, 2)
returns something meaningful, rather than getting stuck in an infinite loop. It is possible to refer to previous parameters in the default value of a parameter.
Reversing an array
Arrays have a reverse
method that changes the array by inverting the society in which its elements appear. For this exercise, write two functions, reverseArray
and reverseArrayInPlace
. The first, reverseArray
, takes an array every bit argument and produces a new array that has the same elements in the changed order. The second, reverseArrayInPlace
, does what the reverse
method does: information technology modifies the assortment given as argument by reversing its elements. Neither may utilise the standard reverse
method.
Thinking back to the notes nigh side effects and pure functions in the previous affiliate, which variant do you expect to be useful in more than situations? Which one runs faster?
console.log(reverseArray(["A", "B", "C"])); allow arrayValue = [i, 2, iii, 4, 5]; reverseArrayInPlace(arrayValue); console.log(arrayValue);
There are two obvious ways to implement reverseArray
. The first is to simply get over the input array from front to dorsum and use the unshift
method on the new array to insert each element at its start. The second is to loop over the input array backwards and use the push button
method. Iterating over an array backwards requires a (somewhat awkward) for
specification, similar (let i = assortment.
.
Reversing the array in place is harder. Yous take to be careful not to overwrite elements that y'all will later need. Using reverseArray
or otherwise copying the whole assortment (array.piece(0)
is a good way to re-create an array) works just is adulterous.
The trick is to bandy the first and last elements, and then the 2nd and second-to-terminal, and and so on. You can do this by looping over one-half the length of the array (use Math.floor
to round down—y'all don't need to touch the middle element in an array with an odd number of elements) and swapping the chemical element at position i
with the one at position array.
. Y'all can use a local bounden to briefly hold on to 1 of the elements, overwrite that one with its mirror epitome, and then put the value from the local bounden in the place where the mirror prototype used to exist.
A list
Objects, every bit generic blobs of values, tin be used to build all sorts of data structures. A common data structure is the list (not to be confused with assortment). A list is a nested prepare of objects, with the get-go object holding a reference to the second, the second to the third, and so on.
let listing = { value: 1, residual: { value: 2, balance: { value: 3, rest: null } } };
The resulting objects form a concatenation, like this:
A dainty thing about lists is that they can share parts of their construction. For example, if I create two new values {value: 0, balance: listing}
and {value: -1, residuum: list}
(with list
referring to the bounden defined earlier), they are both independent lists, but they share the structure that makes upwardly their terminal three elements. The original list is also withal a valid iii-element list.
Write a function arrayToList
that builds up a list construction like the one shown when given [1, 2, 3]
as argument. Also write a listToArray
office that produces an assortment from a list. Then add a helper function prepend
, which takes an chemical element and a listing and creates a new list that adds the element to the forepart of the input list, and nth
, which takes a list and a number and returns the element at the given position in the list (with naught referring to the commencement element) or undefined
when there is no such element.
If you haven't already, also write a recursive version of nth
.
panel.log(arrayToList([ten, 20])); console.log(listToArray(arrayToList([10, 20, 30]))); console.log(prepend(10, prepend(20, null))); panel.log(nth(arrayToList([10, xx, xxx]), 1));
Edifice up a list is easier when done back to forepart. And so arrayToList
could iterate over the assortment backwards (see the previous exercise) and, for each element, add together an object to the listing. You can use a local binding to hold the office of the list that was built so far and use an assignment like listing = {value: 10, residuum: listing}
to add an element.
To run over a list (in listToArray
and nth
), a for
loop specification like this can be used:
for (let node = list; node; node = node.rest) {}
Can you see how that works? Every iteration of the loop, node
points to the current sublist, and the torso can read its value
property to get the electric current element. At the stop of an iteration, node
moves to the next sublist. When that is null, we have reached the end of the list, and the loop is finished.
The recursive version of nth
will, similarly, look at an ever smaller role of the "tail" of the list and at the same time count down the index until it reaches zero, at which signal it can return the value
belongings of the node it is looking at. To get the zeroth chemical element of a list, you simply accept the value
property of its head node. To become element N + 1, you accept the Norththursday element of the list that's in this listing's remainder
holding.
Deep comparison
The ==
operator compares objects by identity. But sometimes you'd prefer to compare the values of their actual properties.
Write a role deepEqual
that takes two values and returns truthful only if they are the same value or are objects with the same properties, where the values of the properties are equal when compared with a recursive phone call to deepEqual
.
To detect out whether values should exist compared directly (use the ===
operator for that) or have their properties compared, you tin use the typeof
operator. If it produces "object"
for both values, you lot should practice a deep comparison. But y'all accept to have 1 silly exception into account: because of a historical accident, typeof null
also produces "object"
.
The Object.keys
function will exist useful when yous need to go over the properties of objects to compare them.
let obj = {here: {is: "an"}, object: ii}; console.log(deepEqual(obj, obj)); console.log(deepEqual(obj, {here: 1, object: 2})); console.log(deepEqual(obj, {here: {is: "an"}, object: 2}));
Your test for whether y'all are dealing with a existent object volition await something like typeof x == "object" && x != null
. Exist careful to compare properties only when both arguments are objects. In all other cases you can just immediately return the issue of applying ===
.
Use Object.keys
to become over the properties. You demand to test whether both objects have the same set of property names and whether those backdrop take identical values. Ane way to do that is to ensure that both objects have the same number of properties (the lengths of the holding lists are the same). And so, when looping over ane of the object'due south properties to compare them, always first make certain the other actually has a property past that name. If they accept the aforementioned number of properties and all properties in 1 as well exist in the other, they accept the same set of property names.
Returning the right value from the function is best done by immediately returning imitation when a mismatch is found and returning true at the end of the part.
makinsonfoughurpite.blogspot.com
Source: https://eloquentjavascript.net/04_data.html
0 Response to "How to Read Value From Array Object in Javascript"
Post a Comment